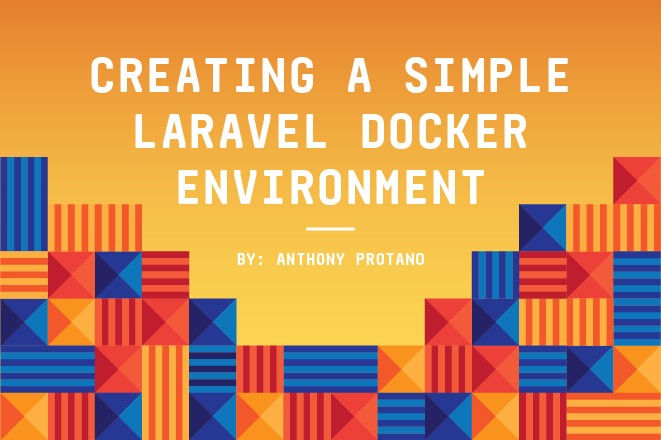
Since I started at liquidfish a little over a year ago I was introduced to the wonderful world of Laravel Homestead. Laravel Homestead is great, it supports multiple php versions, xDebug, MySQL and allows the user to install any other required software straight into the virtual machine.
This is a tried and tested method of local development that has worked incredibly well for our development team for many years, but as we continue to grow we are always looking for ways to improve our development and deployment process, enter docker.
- Docker is a platform for developers and sysadmins to develop, deploy, and run applications with containers. - docs.docker.com
Today we are going to create a dead simple docker environment to run Laravel 5.8 using PHP 7 with xDebug and Mysql on Windows 10 WSL.
My current development environment:
- Windows Subsystem for Linux (WSL)
- My main hard drive is mounted under /c/ in the WSL
- ConEmu using ZSH hooked up to WSL
- Docker for Windows
- Docker & Docker Compose installed on WSL
- PHPSTORM
As long as you have Docker & Docker Compose running on your machine you will be able to follow along with this tutorial. Let’s get started!
First create a new project folder for our docker environment, mine will be under /c/docker/laravel.
Now create the following directories inside the project folder
- docker
- nginx
- php
- config
- www
The docker folder contains three folders, nginx, php, and mysql. These folders will hold Docker related files, Dockerfiles, .inis, configuration files, etc.
The www folder will be our environments web root where laravel will be installed.
First we are going to create a .env file in the root of the project to hold our Docker configuration variables that our compose file and PHP Docker file will use. The .env file will allow for easier flexibility in the event you need to change your environment.
Now let's create our docker-compose. YML file inside the root of our project and add the following services to it.
Docker-compose.yml explanation
Next up is creating our PHP dockerfile
Dockerfile explanation
Now lets create our default configuration files
Create a nginx.conf file in the root of our docker/nginx folder with the following contents.
Create a site.conf file in the root of our docker/nginx/conf.d folder with the following contents. This is a standard laravel nginx config.
I am using test.local as my local domain, be sure to add 127.0.0.1 test.local (or your preferred local domain) to your hosts file.
Create a xdebug.ini file in the root of our docker/php/config folder with the following contents.
The big takeaway with this xdebug configuration is setting connect_back to 0, setting your idekey to PHPSTORM, and setting your hoist to host.docker.internal. I ran into issues with enabling setting remote_connect_back instead of using the remote_host. This has been tested on Windows 10 WSL and has been working really well. If you can’t get xdebug to work with that configuration you may need to search around to find one that will work for you.
Let's build it
To build the environment make sure you are in your projects root folder and run docker-compose build and let it run. When it is done you should see the following output.
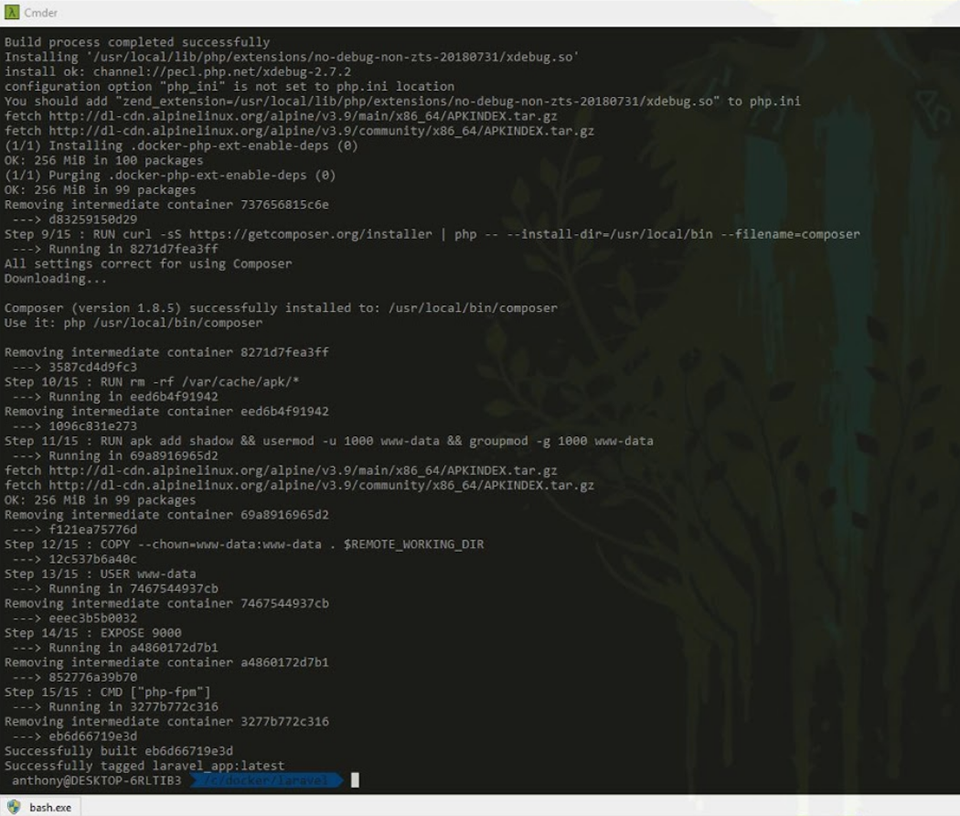
Now that PHP is built lets run docker-compose up -d this command starts all of the containers in detached mode, if you want to be able to run the containers while looking at the output logs just run docker-compose up. If you are in detached mode and want to view the logs for a specific container you can run the following command, docker-compose logs app (container name.)
Installing Laravel
Now that are containers are running lets install laravel, run the following command to jump into the container docker-compose exec app bash.
Now that we are in the container run the following command to install laravel composer create-project --prefer-dist laravel/laravel . Setting the directory to the (.) just means we are going to install laravel inside the current directory.
Great Laravel is installed, now open up test.local (or the domain you set in the nginx config / hosts file) and you will see the Laravel welcome screen!
Setting the laravel mysql environment variables
Just like in the Nginx config where we set the fastcgi_pass to app:9000 we are going to do a similar thing to the mysql environment variables.
Now that we have the environment variables set lets exec back into our container (docker-compose exec app bash) and run the default migrations (php artisan migrate)
Creating a PHP remote debug configuration in PHPstorm
To enable use xdebug in PHPSTORM follow these steps
- Top right of PHPSTORM there is a drop down, click add or edit configuration
- Set the name (Can be anything)
- Select Filter debug connection by IDE key
- IDE Key is PHPSTORM
- Click the servers button to the right of the server dropdown
- Set the name of the server (Can be anything)
- Set the host to your local site url (mine is test.local) Port 80, Debugger Xdebug
- Open the File/Directory selection until you see the www folder
- On the right where it says absolute path on the server click to edit it
- Enter /var/www/html which is the remote path and hit enter on the keyboard to save it
- Apply the changes then hit ok on the servers menu and then the debug menu
To test this go into the web.php routes file and add the following code
Break on the first $word variable and you will now be able to step through the code!
Sweet! Docker is setup, Laravel is installed and configured, our migrations have been ran, and our xDebug configuration for PHPSTORM has been setup!
Now all that's left to do is get building!