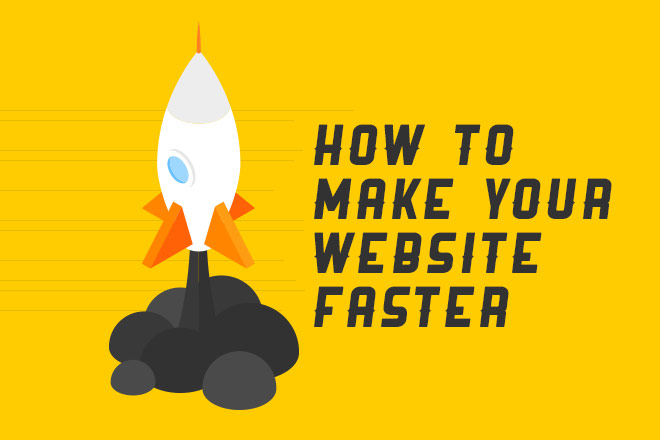
In order for our sites to load quickly, we need to understand what makes them load slowly. Large images, multiple fonts, tons of stylesheets/scripts, render blocking content: When combined, all of these things take a drastic toll on a user’s experience.
Don't block me now
When it comes to assets, we need to understand how they are loaded. Images are non-blocking assets, meaning they do not stop a site from rendering while loading. On the other hand, non-inline stylesheets and scripts are render-blocking assets. These halt a page from loading until the browser fully understands what it needs to do with these styles or functions. So, now that we know what render blocking is, let’s fix the problem.
Loading scripts and stylesheets
When you are loading your stylesheets and scripts, it is recommended to place them after the closing body tag . This will allow all of the content to be rendered, and then the styles and scripts will be interpreted. With critical CSS in place, we will not notice a flash of unstyled content (FOUC). (We will talk about this in the next section.).
Another solution that has recently surfaced is to include these stylesheets and scripts with JavaScript so they are loaded as non-blocking assets. When assets are added dynamically like this, they are executed outside of document parsing, so rendering isn’t blocked while they’re downloading.
In non-developer terms, the above code takes the provided URL's and creates a script or style tag dynamically for them. It then inserts the tag into the head of the document and loads it's contents.
Critical CSS ftw
If you have ever opened up Inspector and found a style tag in the head with tons and tons of styles, you have probably found a hidden gem of optimization. Critical CSS is the CSS needed to render the “above the fold” content of your site. The fold doesn’t really exist on the Web nowadays, so I tend to pick a general browser size to generate the critical CSS.
When I first read about this idea, I was immediately turned off by it due to not wanting to hand pick each line of code that needs to be included in the head of the document. Luckily for us, there are build tools out there that will help ease the pain. If you are using Grunt, there is a plugin called criticalcss by Filament Group. With a few lines of code, you can have the plugin create a file with the critical CSS needed for each page. I won’t go into details about how it works, but definitely check out the GitHub repo.
One last tip I have begun to implement is inlining my font stylesheet. This prevents FOUC, which happens when the font stylesheet is loaded after the body or via async.
Make sure you minify all of your CSS and JS!
More images, more problems
Images are great to use; they can really bring a site to life. Using images carelessly, however, can damage your creation. The most important thing to remember when adding images is to optimize them as much as possible.
You can optimize your images however you like. Photoshop allows you to set the level of compression when you save an image for the Web. There are also free online resources to accomplish this as well. TinyPNG, JPEG Optimizer and Compressor.io are just a few that come to mind.
Again, there is a Grunt plugin that can do this as well, called imagemin. It will watch a specified folder for any new images and optimize them once they are added. It will save out the new image to another folder if needed so the file is not overwritten.
The optimization levels will need to be determined on an image-by-image basis. When saving for the Web via Photoshop, I tend to set the level between 45 and 65, depending on how it looks compared to the original.
Overview
This isn’t a complete guide to make your site the fastest out there, but these steps will definitely make an improvement and help you get started with site optimization. Inlining your critical CSS and loading your assets dynamically will effectively boost your site’s performance; Go ahead and try it out, you’ll see. Optimizing your images just right will bring their file size down without noticing a loss in quality. This can be tricky, but with the mentioned tools and techniques it becomes quite painless.
If you are wanting to test just how fast your site is performing, check out Google’s PageSpeed Insights and WebPageTest’s performance tool. Try to aim for a 100/100 on PageSpeed Insights and 1,000 speed index on WebPageTest if you need a benchmark to go off of.