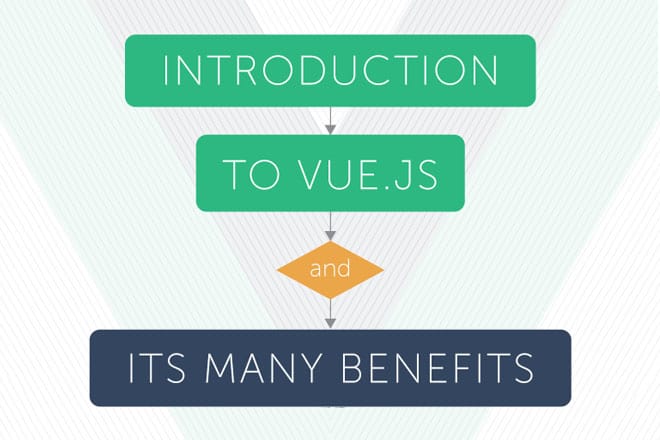
I recently had the opportunity to add a new tool to my development toolbox in the form of Vue.js. The Vue.js documentation calls it “a library for building interactive web interfaces,” but thinking of it only in interface terms would be underselling it. I think of it more as library that makes it incredibly easy to transform data into html. An added bonus is that the page is updated automatically if your data happens to change.
How does Vue.js work?
Let's look at an example. Maybe you have a list of products you want to display on the page and the data looks something like this:
For vue.js to work (and I’m pretty sure there is some magic involved here) you need two things: a vue.js instance and template to which the instance is bound. In our example, assuming the vue.js source has already been included in the page, we only need a tiny snippet of script which should be run when the page DOM is ready:
This one little line does three important things: it creates a vue.js instance, it binds that instance to the DOM element with the ID “products,” and it binds the product_list array created above to its internal variable “products”. The only thing we’re missing now is a place to display the data.
A vue.js template for this might look something like this:
Where does the Vue.js template go?
It is included directly in your page wherever you want the output to appear. We see a couple of unusual attributes in here, but even without being familiar with vue.js, what’s going on should be fairly clear. The “v-for” directive is simple for loop. Notice how were are able to nest these as we drill down into the data. We also see how vue allows us to use our data to define styles using the “v-bind:style” directive. Of course, the double curly brackets denote where view will insert values from the data. These two directives merely scratch the surface of what vue.js is capable of.
Why use vue.js?
If all we wanted to do was render a list on the page, loading an extra library wouldn’t be very efficient; the cool thing is that if the data changes, the page is updated immediately. If you push a new product object into the product_list array, vue.js will work its magic and the new product will be added to the page DOM almost immediately. You can even completely replace the data and vue will rerender the template using this new data. Of course, vue.js is capable of so much more than what I have illustrated in this simple example. You can add conditionals, event handlers, have multiple vue instances on a page, create reusable components, and much more. Check out Vuejs.org for more information and to add this framework to your toolbox.